Circuit Connection
Using Arduino to control a Sabertooth motor driver is very easy. Please don't get confused with the multiple modes that a Sabertooth driver provides you. The following picture demonstrates an easy connection.- I used an Arduino Mega 2560
compatible board, and placed an IO
expansion shield on top of the Arduino. I used a Sabertooth2X12
board.
- Use any PWM pin that the Arduino provides, here I used pin 14.
Connect pin 14 to the S1 slot of the Sabertooth to send PWM signal to
the first motor (A Sabertooth is capable of controlling two motors,
here I only connected one motor).
- Connect a ground pin from Arduino to the 0V slot of the Sabertooth to provide ground reference volt.
- Connect two wires from the power supply to B+ and B- slots on
Sabertooth to provide power to the motor.
- Connect the two wires from the motor to M1A and M1B slots on Sabertooth to allow current to flow from your power supply to your motor.
- On Sabertooth, use the Microcontroller
pluses, independent linear control mode. On Sabertooth 2X12,
this means 1, 4, 6 down, 2, 3, 5 up.
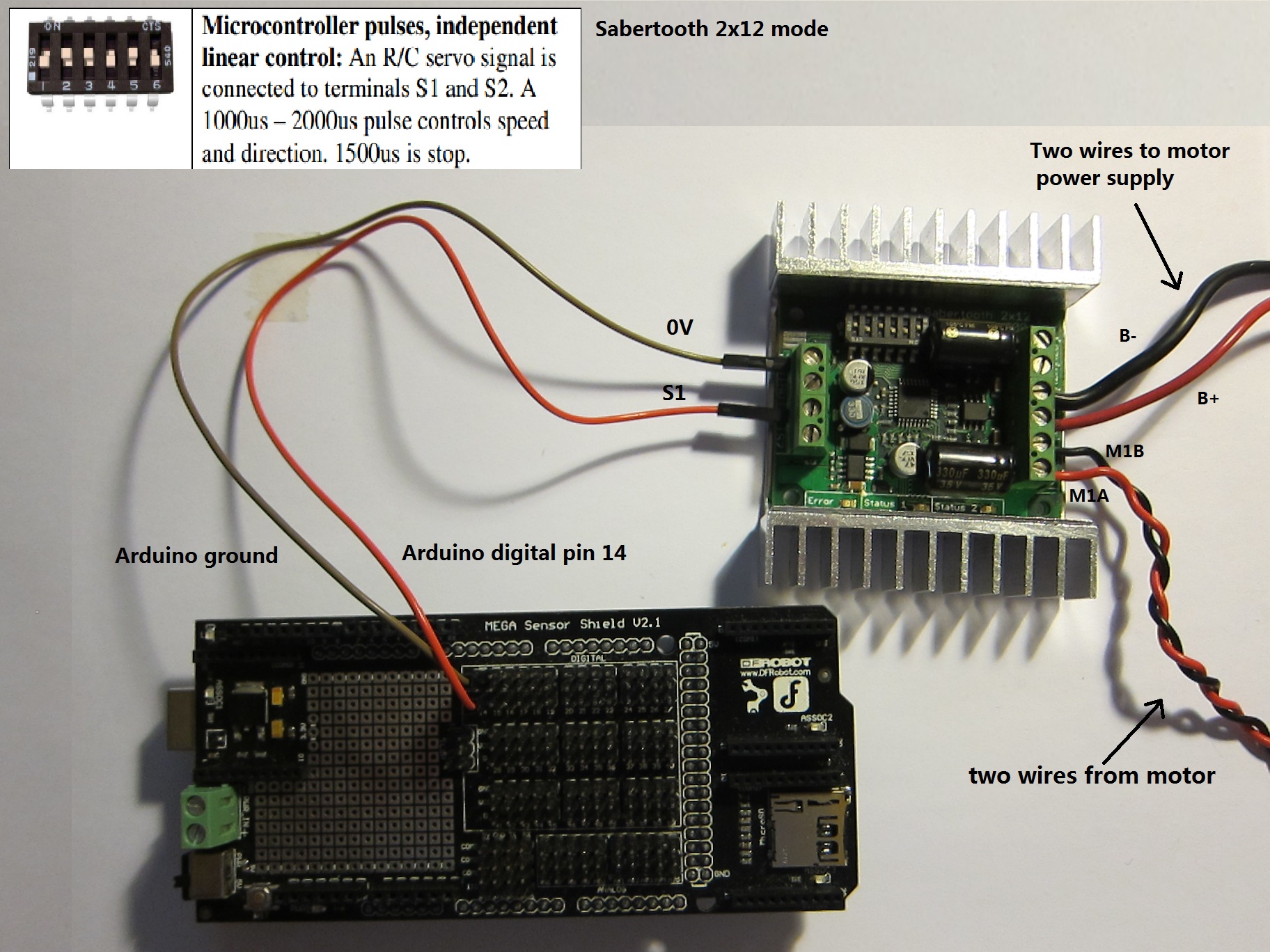
Arduino Program
The Arduino program to control the motor is also very simple by using the Servo library:#include <Servo.h>
Servo myservo;
void setup () {
myservo.attach(14); // Use PWM pin 14 to control Sabertooth.
}
void loop() {
// 0 means full power in one direction.
// Actually the minimum value for me is around 30.
// A smaller value won't drive the motor.
myservo.write(30);
delay(2000);
// 90 means stopping the motor.
myservo.write(90);
delay(2000);
// 180 means full power in the other direction.
// Actually the maximum value for me is around 160.
// A larger value won't drive the motor either.
myservo.write(160);
delay(2000);
}
Servo myservo;
void setup () {
myservo.attach(14); // Use PWM pin 14 to control Sabertooth.
}
void loop() {
// 0 means full power in one direction.
// Actually the minimum value for me is around 30.
// A smaller value won't drive the motor.
myservo.write(30);
delay(2000);
// 90 means stopping the motor.
myservo.write(90);
delay(2000);
// 180 means full power in the other direction.
// Actually the maximum value for me is around 160.
// A larger value won't drive the motor either.
myservo.write(160);
delay(2000);
}